JS原生实现简单ajax请求
一、写个简单的HTML页面
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>Ajax请求测试</title>
<link rel="stylesheet" href="http://at.alicdn.com/t/font_2335994_6js183z4xv2.css">
<script src="./js/jquery-3.1.1.js"></script>
</head>
<body>
<div class="container">
<div class="search">
<input type="text" placeholder="请输入关键字查询">
<button>
<i class="iconfont icon-sousuo"></i>
搜索
</button>
</div>
<div class="search-info">
<ul class="search-list"></ul>
</div>
</div>
</body>
</html>
二、随便写点CSS
<style>
*{
margin:0;
padding:0;
box-sizing: border-box;
}
li{
list-style:none;
}
a{
text-decoration:none;
}
.container{
width: 800px;
height: auto;
background-color:#f2f2f2;
margin:50px auto;
overflow: hidden;
}
.search{
width: 600px;
height: 50px;
line-height: 50px;
background-color:#228b22;
margin:50px auto auto;
display: flex;
flex-direction:row;
justify-content: space-between;
}
.search input{
flex:10;
border:1px solid #228b22;
border-right:none;
outline:none;
text-indent:20px;
}
.search button{
flex:2;
font-size: 14px;
color:#228b22;
border:1px solid #228b22;
outline:none;
background-color: #fff;
}
.search-info{
display:none;
width:600px;
height:auto;
background-color:#fff;
margin:0 auto;
border:1px solid #228b22;
border-top:none;
}
ul{
padding:20px;
}
ul > li{
margin:0 0 10px 0;
font-size:14px;
line-height: 14px;
color:#666666;
}
</style>
三、JS原生实现Ajax
问:这不是用了JQuery吗,扯什么原生?
答:DOM元素的处理使用了JQuery,因为更方便,只是为了简化JS操作DOM的步骤,AJAX还是JS原生的
<script>
//获取页面中的输入框元素并绑定oninput和onpropertychange事件,实现对input内容的实时监控
$('input').bind('input propertychange', function(){
if(!($('input').val()=="")){
//初始化xhttp对象为null
let xhttp = null;
if(window.XMLHttpRequest){
//现代浏览器的创建XMLHttp对象的方式(IE7+)
xhttp = new XMLHttpRequest();
}else{
//非现代浏览器创建XMLHttp对象的方式(IE7-)
xhttp = new ActiveXObject("Microsoft.XMLHTTP");
}
//判断xhttp对象获取成功,执行以下操作
if(xhttp!=null){
// 监听ajax请求就绪事件
xhttp.onreadystatechange = function(){
// 请求就绪,响应正确,就开始解析返回的数据
if(xhttp.readyState===4 && xhttp.status===200){
// 将返回的字符串数据解析为JSON对象
let responseData = JSON.parse(xhttp.responseText);
// 每次重新请求的同时,移除列表中所有li元素
$('.search-list').find("li").remove();
for (const filminfo of responseData) {
// 向ul列表中追加子元素li
$('.search-list').append("<li class=\"list-item\">"+filminfo["name"]+"</li>");
console.log("<li class=\"list-item\">"+filminfo["name"]+"</li>");
}
// 由上到下展开显示列表
$('.search-info').slideDown(300);
}
}
// 建立异步请求的链接
xhttp.open("GET","/360Video/demo1?keyword="+$('input').val(),true);
// 发送异步请求
xhttp.send();
}
}
});
// 输入框失焦,隐藏列表
$('input').blur(function(){
$('.search-info').slideUp(300);
});
</script>
四、写个简单的Servlet去响应ajax请求
@WebServlet(urlPatterns = "/demo1")
public class ServletDemo1 extends HttpServlet {
@Override
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
this.doGet(request, response);
}
@Override
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
String keyword = request.getParameter("keyword");
System.out.println(keyword);
// 这里我使用自己的工具类去拿了360影视的数据,以json格式返回
String data = HttpUtils.getData("https://www.360kan.com/dianying/list.php?rank=rankhot&cat=all&area=all&act=all&year=all&pageno=2&from=dianying_list");
// 这里必须要这么设置Content-Type,不然前端获取到的json里面中文是乱码的
response.setContentType("application/json;charset=UTF-8");
// 将json数据写出到页面
response.getWriter().write(data);
}
}
五、最终效果展示
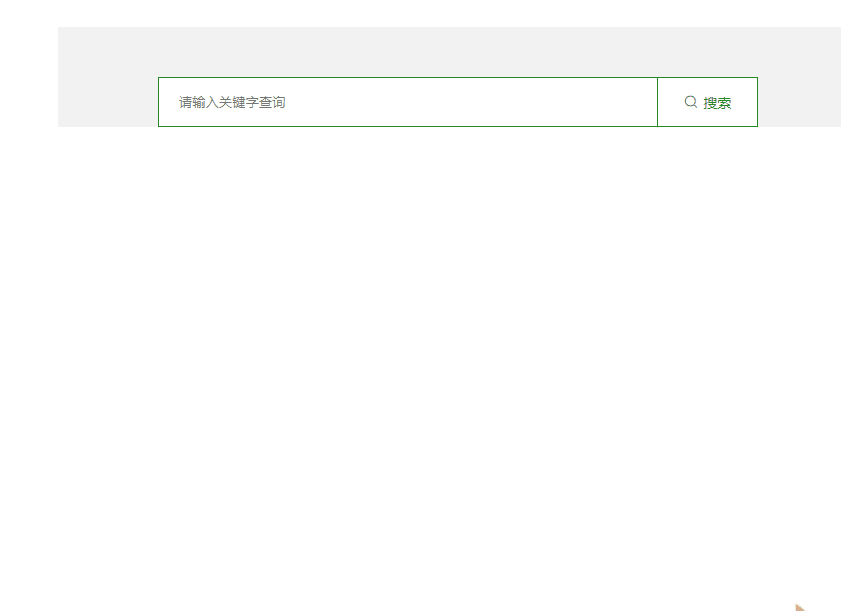
原文作者:絷缘
作者邮箱:zhiyuanworkemail@163.com
原文地址:https://zhiyuandnc.github.io/3g0TLSILu/
版权声明:本文为博主原创文章,转载请注明原文链接作者信息